Every BI Publisher exposes its web service URL. The pattern of the URL is as below:
http://[Host]:[Port]/xmlpserver/services/PublicReportService?WSDL
Part 1: Creating BIP Web Service Client in JDev application.
Pre-Requisite:
Fusion Web Application project is created and BIP is available.
1. Right
click on Model project and select New
2. Select
the Web Service from Business Tire components and select Web Service Proxy.
3. Click Next
4. Select JAX-WS Style and click Next
5. At
WSDL Document URL put the BIP Web Service URL and click Next
6. Enter
the package name as stated below and click Next
7. Click
Next
8. Leave
the current selection and click Next
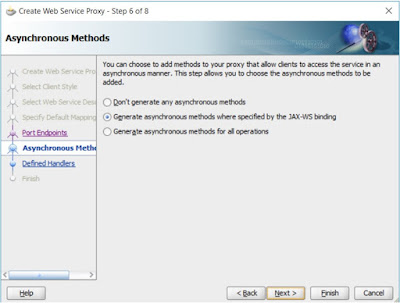
10. Leave
the existing setup and Click Next
11. Click
on Finish
12. Once
it is finished, it will look like below.
We will
be using the PublicReportServiceClient.java under com.bip.client to generate
report from BI Publishers. All other classes will be used internally.
Part 2: Generate Report using Java
Pre-Requisite: BIP Client is
created and sample report is present in BI Publisher
Open the class PublicReportServiceClient from PublicReportServiceClient.java
Initially it will look like below:
We need
to use this class and change it accordingly.
First we need to authenticate and create a new session in the connected BIP. In order to achieve that, use the below snippet.
//the URL I used, so that I can connect to any BIP from here. It
doesnt matter for which BIP you created the client.
URL url
= new URL("http://test.abcd.com:9704/xmlpserver/services/PublicReportService");
QName qname = new QName("http://xmlns.oracle.com/oxp/service/PublicReportService","PublicReportServiceService");
//Creating client class for the above
mention BIP URL.
publicReportServiceService
= new PublicReportServiceService(url,qname);
PublicReportService publicReportService =
publicReportServiceService.getPublicReportService();
//connect,
Login and creating new seesion to the BIP
String
sessionId = publicReportService.login([USERNAME], [PASSWORD]");
System.out.println("sessionId : "+sessionId);
|
Then create a ReportDefinition object to get all the input parameter of a Report, which will be generated by this code. Run the code and get the input parameter name. Use this parameter to set value for the report.
//We need to get the name of the input parameter.
//So we are creating the report definition of a specific report to
get the details, like inpot parameter.
ReportDefinition reportDef =
publicReportService.getReportDefinitionInSession("/Oracle Identity Manager/Oracle Reports/Access Policy Reports/Access Policy Details.xdo", sessionId);
ArrayOfParamNameValue valArray =
reportDef.getReportParameterNameValues();
//get all
the associated input parameter from the below code.
// This will be
required to generate report based on input parameter.
ArrayOfString colArray = reportDef.getParameterNames();
for(int i=0; i<colArray.getItem().size(); i++){
System.out.println("Cols
: "+colArray.getItem().get(i));
}
|
Set the input parameter value for the report
//Setting
input parameters for the report. in this case I am using
//the OIM
Entitlement name to get the current access list report
ArrayOfString values = new ArrayOfString();
values.getItem().add("");
|
Create ReportRequset object
//Creating
ReportRequest object and set required values
ReportRequest req = new ReportRequest();
req.setAttributeFormat("pdf"); //type of the report
req.setAttributeLocale("en-US"); //Language
req.setAttributeTemplate("Simple"); //Templet type
req.setReportAbsolutePath("/Oracle Identity Manager/Oracle Reports/Access Policy Reports/Access Policy Details.xdo");
//Absolute path of the report from BIP Catalog.
req.setSizeOfDataChunkDownload(-1); //to download all
req.setParameterNameValues(valArray);
|
Create ReportResponse object, where ReportRequest object will be used to generate report.
//Create
ReportResponse object to create report based on ReportRequest object.
ReportResponse res = new ReportResponse();
res = publicReportService.runReportInSession(req, sessionId);
byte[] binBytes = res.getReportBytes(); //Generated report stored in a
byte[]
|
Save the report in pdf file.
//Saving
the report in a pdf file.
FileWriter fstream = new
FileWriter("E:\\export_loc\\BIP\\testReport.pdf");
OutputStream out = new
FileOutputStream("E:\\export_loc\\BIP\\testReport.pdf");
out.write(binBytes);
//closing
the output stream
out.close();
|
Full Code
package com.bip.client;
import com.bip.service.ArrayOfParamNameValue;
import com.bip.service.ArrayOfString;
import com.bip.service.ReportDefinition;
import com.bip.service.ReportRequest;
import com.bip.service.ReportResponse;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.OutputStream;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.WebServiceRef;
// !THE CHANGES MADE TO THIS FILE WILL BE DESTROYED IF
REGENERATED!
// This source file is generated by Oracle tools
// Contents may be subject to change
// For reporting problems, use the following
// Version = Oracle WebServices (11.1.1.0.0, build
111209.0821.28162)
public class PublicReportServiceClient
{
@WebServiceRef
private static PublicReportServiceService publicReportServiceService;
public static void main(String [] args)
{
try
{
//the URL I
used, so that I can connect to any BIP from here. It doesnt matter for which
BIP you created the client.
URL url = new
URL("http://test.abcd.com:9704/xmlpserver/services/PublicReportService");
QName qname = new
QName("http://xmlns.oracle.com/oxp/service/PublicReportService","PublicReportServiceService");
//Creating
client class for the above mention BIP URL.
publicReportServiceService = new PublicReportServiceService(url,qname);
PublicReportService publicReportService =
publicReportServiceService.getPublicReportService();
//connect,
Login and creating new seesion to the BIP
String sessionId = publicReportService.login("username",
"Password");
System.out.println("sessionId : "+sessionId);
//Setting
input parameters for the report. in this case I am using
//the OIM
Entitlement name to get the current access list report
ArrayOfString values = new ArrayOfString();
values.getItem().add("Check Testing_Sample_POC");
//value of the input parameter
//We need
to get the name of the input parameter.
//So we are
creating the report definition of a specific report to get the details, like
input parameter.
ReportDefinition reportDef =
publicReportService.getReportDefinitionInSession("/Oracle Identity
Manager/Oracle Reports/Access Policy Reports/Access Policy Details.xdo",
sessionId);
ArrayOfParamNameValue valArray =
reportDef.getReportParameterNameValues();
//get all
the associated input parameter from the below code.
// This will be
required to generate report based on input parameter.
ArrayOfString colArray = reportDef.getParameterNames();
for(int i=0; i<colArray.getItem().size(); i++){
System.out.println("Cols
: "+colArray.getItem().get(i));
// Setting
value for the input parameter
//p_varchar_PolicyName is the one of the input parameter got from the
above System.out.println("Cols
: "+colArray.getItem().get(i));
if (valArray.getItem().get(i).getName().equals("p_varchar_PolicyName")){
valArray.getItem().get(i).setValues(values);
}
}
//Creating
ReportRequest object and set required values
ReportRequest req = new ReportRequest();
req.setAttributeFormat("pdf"); //type of
the report
req.setAttributeLocale("en-US"); //Language
req.setAttributeTemplate("Simple"); //Template
type
req.setReportAbsolutePath("/Oracle Identity
Manager/Oracle Reports/Access Policy Reports/Access Policy
Details.xdo"); //Absolute path of the report from BIP Catalog.
req.setSizeOfDataChunkDownload(-1); //to
download all
req.setParameterNameValues(valArray);
//Create
ReportResponse object to create report based on ReportRequest object.
ReportResponse res = new ReportResponse();
res = publicReportService.runReportInSession(req, sessionId);
byte[] binBytes = res.getReportBytes(); //Generated report
stored in a byte[]
//Saving
the report in a pdf file.
FileWriter fstream = new
FileWriter("E:\\export_loc\\BIP\\testReport.pdf");
OutputStream out = new FileOutputStream("E:\\export_loc\\BIP\\testReport.pdf");
out.write(binBytes);
//closing
the output stream
out.close();
} catch (MalformedURLException murle) {
// TODO: Add catch code
murle.printStackTrace();
}catch (AccessDeniedException ade) {
// TODO: Add catch code
ade.printStackTrace();
}catch (InvalidParametersException ipe) {
// TODO: Add catch code
ipe.printStackTrace();
} catch (OperationFailedException ofe) {
// TODO: Add catch code
ofe.printStackTrace();
}catch (Exception e) {
// TODO: Add catch code
e.printStackTrace();
}
}
}
|
Run the code and generate the report
thank you so much. .
ReplyDeleteI got my reports
Can you please tell me how to schedule BIP reports through web Services
ReplyDeleteHi..Good to hear that u are able to generate BIP reports through APIs. I have seen the APIs available for triggering schedule job, but haven't seen anything to create schedule job. U can check theis URL https://docs.oracle.com/cd/E24001_01/bi.1111/e18863/scheduleservice.htm#CIHEEFII
DeleteHi ,I have coming to ur blog it's very committed to knowledge, great work,,please help me as in ,I have urgent requirement for scheduling report using BIP web services through Java in Adf,, please any help would be appreciated
ReplyDelete